Aktywne Wpisy
Zawiera treści 18+
Ta treść została oznaczona jako materiał kontrowersyjny lub dla dorosłych.

JakDorobic_com +101
W piątkowy (prawie) wieczór proponuję świeżutkie, pachnące… #rozdajo (╭☞σ ͜ʖσ)╭☞
Do wygrania jeszcze raz zaproponujemy Wam przysmak wszystkich #mikrokoksy, czyli odżywkę białkową, a konkretnie Izolat białka WPI ISO Core od FA Nutrition (500 gramów), smak CZEKOLADOWY – o wartości rynkowej około 60 zł!
Białko możecie sami spożyć, dać komuś na prezent lub sprzedać z zyskiem! Data przydatności to listopad 2024 rok.
…żeby wziąć
Do wygrania jeszcze raz zaproponujemy Wam przysmak wszystkich #mikrokoksy, czyli odżywkę białkową, a konkretnie Izolat białka WPI ISO Core od FA Nutrition (500 gramów), smak CZEKOLADOWY – o wartości rynkowej około 60 zł!
Białko możecie sami spożyć, dać komuś na prezent lub sprzedać z zyskiem! Data przydatności to listopad 2024 rok.
…żeby wziąć
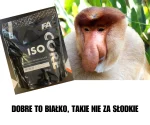
źródło: Szary Lato Dzieci Zabawa Wspomnienia Post na Facebooku
Pobierz
Mam pytanie. Posiadam taką klasę pomocniczą do testów:
@Component
public class SessionChecker {
private final DataSource dataSource;
public SessionChecker(DataSource dataSource) {
this.dataSource = dataSource;
}
public boolean checkExistence(String sessionId) {
try {
String query = "SELECT COUNT(*) FROM sessions WHERE session_id = ?";
try (Connection connection = dataSource.getConnection();
PreparedStatement statement = connection.prepareStatement(query)) {
statement.setString(1, sessionId);
ResultSet resultSet = statement.executeQuery();
return resultSet.next() && resultSet.getInt(1) > 0;
}
} catch (SQLException e) {
throw new RuntimeException("Error during checking if session exists " + e.getMessage(), e);
}
}
public boolean userHasActiveSessions(int userId) {
try {
String query = "SELECT COUNT(*) FROM sessions WHERE user_id = ?";
try (Connection connection = dataSource.getConnection();
PreparedStatement statement = connection.prepareStatement(query)) {
statement.setInt(1, userId);
try (ResultSet resultSet = statement.executeQuery()) {
return resultSet.next() && resultSet.getInt(1) > 0;
}
}
} catch (SQLException e) {
throw new RuntimeException("Error during checking if user has active sessions: " + e.getMessage(), e);
}
}
}
Czy zamiast tych dwóch metod mogę napisać to tak? Taki zapis będzie dobry?
@Component
public class SessionChecker {
private final DataSource dataSource;
public SessionChecker(DataSource dataSource) {
this.dataSource = dataSource;
}
public boolean sessionExist(String sessionId) {
String query = "SELECT COUNT(*) FROM sessions WHERE session_id = ?";
return checkExistence(query, sessionId);
}
public boolean userHasActiveSession(int userId) {
String query = "SELECT COUNT(*) FROM sessions WHERE user_id = ?";
return checkExistence(query, userId);
}
private boolean checkExistence(String query, Object value) {
try (Connection connection = dataSource.getConnection();
PreparedStatement statement = connection.prepareStatement(query)) {
if (value instanceof Integer) {
statement.setInt(1, (Integer) value);
} else if (value instanceof String) {
statement.setString(1, (String) value);
}
ResultSet resultSet = statement.executeQuery();
return resultSet.next() && resultSet.getInt(1) > 0;
} catch (SQLException e) {
throw new RuntimeException("Error during checking existence: " + e.getMessage(), e);
}
}
}
Można to zrobić jakoś lepiej?
Zamiast tego na Twoim miejscu celowałbym w sygnaturę
private boolean checkExistence(String query, PreparedStatement statement)
. Wtedy każda z publicznych metodcheckExistence(String query, Consumer<PreparedStatement> propertySetter) {
try (Connection connection = dataSource.getConnection();
PreparedStatement statement = connection.prepareStatement(query)) {
propertySetter.accept(statement);
ResultSet resultSet = statement.executeQuery();
return resultSet.next() && resultSet.getInt(1) > 0;
} catch (SQLException e) {
throw new RuntimeException("Error during checking existence: " + e.getMessage(), e);
}
public boolean userHasActiveSession(int userId) {
String query = "SELECT COUNT(*) FROM sessions WHERE user_id = ?";
return checkExistence(query, st -> st.setInt(1, userId));
}
public boolean sessionExist(String sessionId)